HackerRank - Climbing the Leaderboard
An arcade game player wants to climb to the top of the leaderboard and track their ranking. The game uses Dense Ranking, so its leaderboard works like this:
The player with the highest score is ranked number 1 on the leaderboard.
Players who have equal scores receive the same ranking number, and the next player(s) receive the immediately following ranking number.
In the above statement two parameters are given:
1st is ranked that is score board.
2nd is player that is Player's score
We have to find the rank that a player receives according to scores, we need to follow two rules.
The player with high score will rank 1 on scoreboard
The player with same score will get same rank.
Let's understand with example, suppose we have ranked with values 100 100 50 40 40 20 10 and player with values 5 25 50 120
ranked :

player :
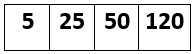
Now Consider the 1st player score 5 in the tournament, 5 is the most smallest score in the scoreboard. So it will get last rank that is 6.
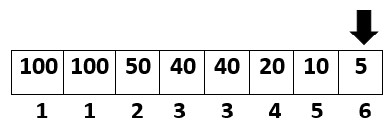
Player Score 25 is greater then 20 and less then 40 so it will be rank 4.
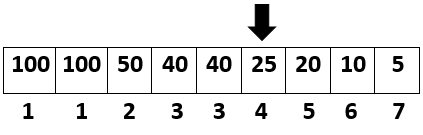
In a same way 50 and 120 will get rank 2 and 1 accordingly.
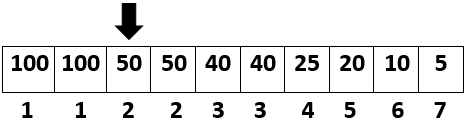
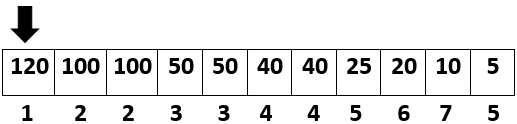
So the rank which player receives are 6,4,2,1 respectively.
function climbingLeaderboard(ranked, player) { ranked = Array.from(new Set(ranked)); // Remove duplicate scores let result = []; let j = ranked.length - 1; // Index of the last score for (let i = 0; i < player.length; i++) { while (j >= 0 && player[i] >= ranked[j]) { j--; // Move to the previous score if player's score is greater } result.push(j + 2); // Add 2 to convert from 0-based index to 1-based rank } return result; }
In the above code we remove all duplicate values from rank as same score will get same rank.
We had titrate each player score and compare it with score in ranked from last until Player's score is greater then rank's score and decrement 'j' variable's value. Once we find player's score is less them rank's score we will push the j+2 in result array (1 for converting 0 index base to 1 base and 1 because we have to add player's rank next to the rank's score which we are comparing).
At last we will return result array.