Diagonal Difference
Given a square matrix, calculate the absolute difference between the sums of its diagonals.
A diagonal is a line segment on a polygon or polyhedron. Diagonals connect two vertices (points, or corners) that do not share a side.
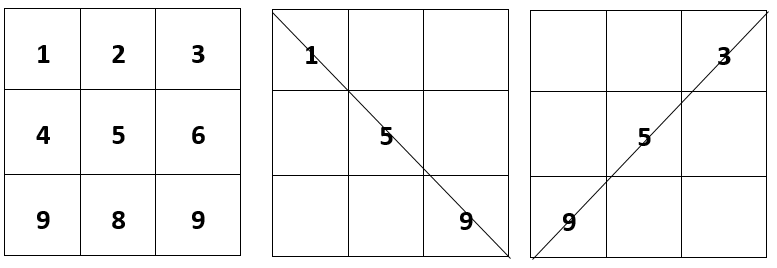
In the above 3X3 matrix 1,5,9 are on the line segment that connects top left corner and bottom right corner.
In a same way 3,5,9 are on the line segment that connect top right and bottom left corner.
So, 1,5,9 and 3,5,9 are diagonals
Now, according to problem statement we need to find the absolute difference between both diagonals
So, 1st we will add both diagonals i.e 1+5+9 = 15 and 3+5+9 = 17
And afterwards we will subtract 2nd Diagonal from 1st Diagonal
function diagonalDifference(arr) { let diagonal1 = 0; let diagonal2 = 0; const n = arr.length; for (let i = 0; i < arr.length; i++) { diagonal1 += arr[i][i]; diagonal2 += arr[i][n - 1 - i]; } return Math.abs(diagonal1 - diagonal2); }
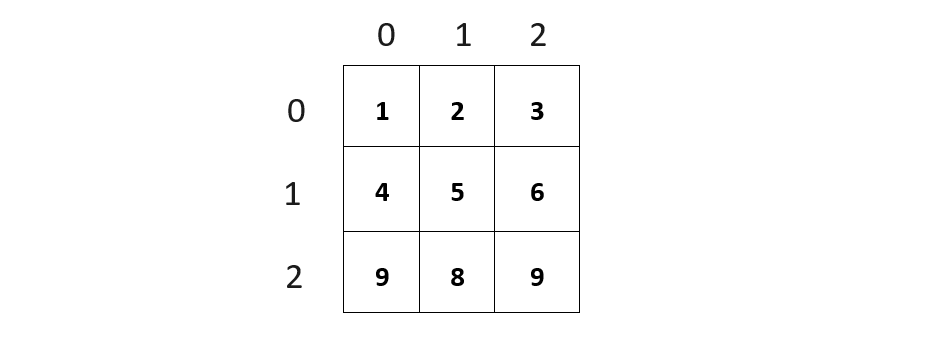
In the above code we itterate each row of the matrix
Diagonal1 is present on arr[i][i] i.e :
arr[0][0] = 1
arr[1][1] = 5
arr[2][2] = 9
Diagonal2 is present on arr[i][n-1-i] i.e :
arr[0][3-1-0] = arr[0][2] = 3
arr[1][3-1-1] = arr[0][1] = 5
arr[2][3-1-1] = arr[2][0] = 9
as we need absolute difference we had use Math.abs()