Constants
The Constants are the values which never changes during the execution of program e.i the value of pi is fixed that is 3.14 which never changes. The C uses following type of constants.
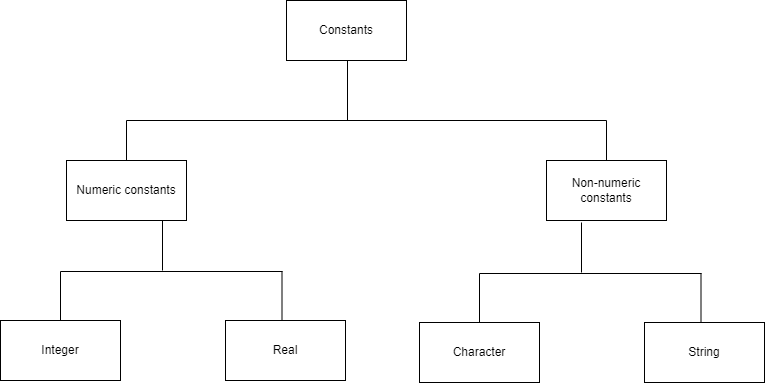
Integer constants :
Integer constants are use to store the whole integer numbers. It Stores digits from 0 to 9 without decimal points and it can be positive(+) or negative(-). Space and special characters like `!@#$%^&*().,'; are not allowed The following are the valid integer constants.
12
-99
1204
0
+5
The C also allows the octal and hexadecimal integer constants in addition to decimal constants. The octal numbers are written with preceding 0 and hexadecimal numbers are preceded by 0x or 0X. The following are examples of valid octal and valid hexadecimal constants.
Octal | Hex |
0177 | 0x32ab |
05 | 0x92f |
0831 | 0x1234 |
For unsigned integer constants, number is followed by 'u' or 'U'. For long integer constants, number is followed by 'l' or 'L'. consider the following examples.
1234u or 1234U | (Unsigned integer) |
123456l or 123456L | (long integer) |
5432178ul or 5432178UL | (unsigned long integer) |
Real constants :
As name suggest real constant are use to store real numbers. Any number positive or negative ,number with decimal points or without decimal points are consider as real number. The following are examples of valid real constants.
3.14
-3.14
+120.0
260.
.90
Real constants can be written in scientific formats also. For example, 314.28 is written in scientific format as 3.1428e2. The following are examples of real constants in scientific format.
638e+4
-5.52E-1
+1.72e+2
8.1E3
The scientific format is normally used to represent the numbers which are too small or too big. For example, 0.000000587 is very small number and written in scientific format as 5.87e7. Similarly, 15000000000 is too big and written in scientific format as 1.5E10.
Character constants :
Character constants are use to store single character. It is enclosed between single quotes(''). we can not store more then one character in it and can not use double quotes in character constants. these character are stored in memory in the form of ASCII (American standard code for information interchange) value. ASCII is a standard format for encoding data in electronic communication. Every key on keyboard has a specific ASCII value i.e ASCII value of 'a' is 97. The following are examples of valid character constants.
'S' 'u' '_' ' C' '10'
Example 2
Write a program to print the ASCII value of a given character.
#include <stdio.h> void main(){ clrscr(); /*use to clear the output screen*/ char ch; printf("Enter a character : "); scanf("%c",&ch); printf("ASCII of \'%ch\' is %d \n",ch,ch); getch(); /*use to hold the output screen as it ask for input of one character*/ }
Result :
-------------------------------
Enter a character : A
ASCII of 'A' is 65
-------------------------------
The C language also uses the special characters known as Escape sequence in addition to normal printable characters. The Escape sequence always starts from the backslash(\) character. We have earlier already used the newline character which is also an Escape sequence represented as '\n' to move the cursor to the next line. Bellow table shows all the Escape sequences and their meaning. These are used normally as output functions.
Constants | meaning |
'\a' | bell |
'\b' | back space |
'\f' | form feed |
'\n' | new line |
'\r' | carriage return |
'\t' | horizontal tab |
'\v' | vertical tab |
'\" | single quote |
'\"' | double quote |
'\?' | question mark |
'\\' | backslash |
'\0' | null |
String constants :
String constant is a sequence of character constants and enclosed in double quotes. The strings are used to represent the names, addresses, city names etc. The following are the examples of valid string constants.
"SUCHCODES"
"2024"
"D"
"Hello, world"
Note that "D" and 'D' are not same. Similarly, "5", '5' and 5 are different.